Problem.
I had a shape, say a paper.js Circle that I wanted to divide into multiple parts, based on cut marks. Cut marks are basically lines which overlap the shape.
The following is an example.
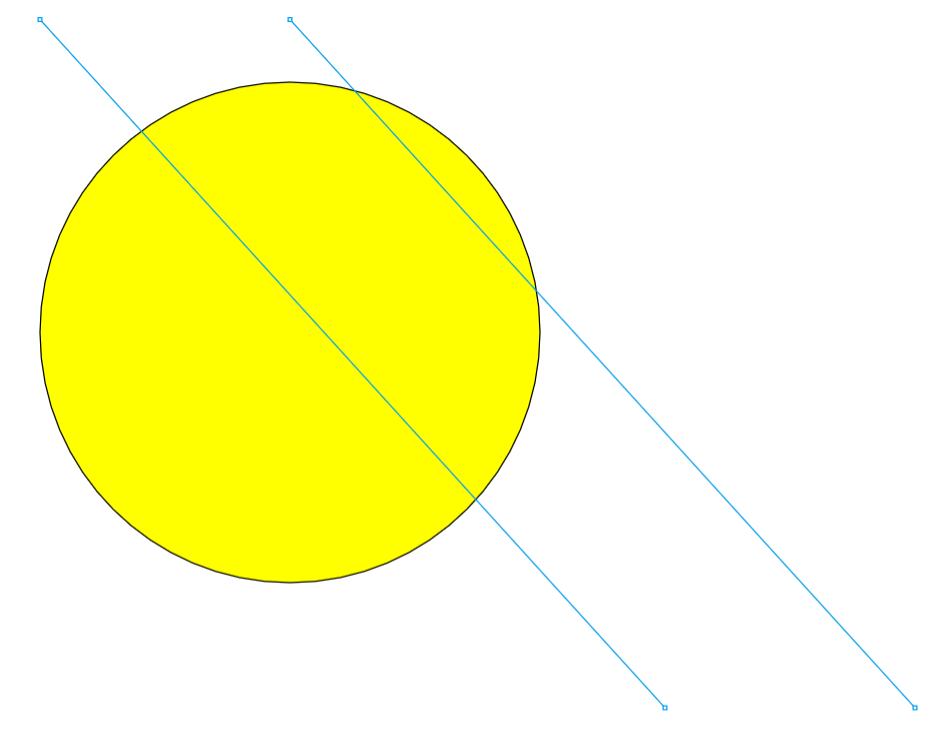
Once I split the shapes they should look like the following.
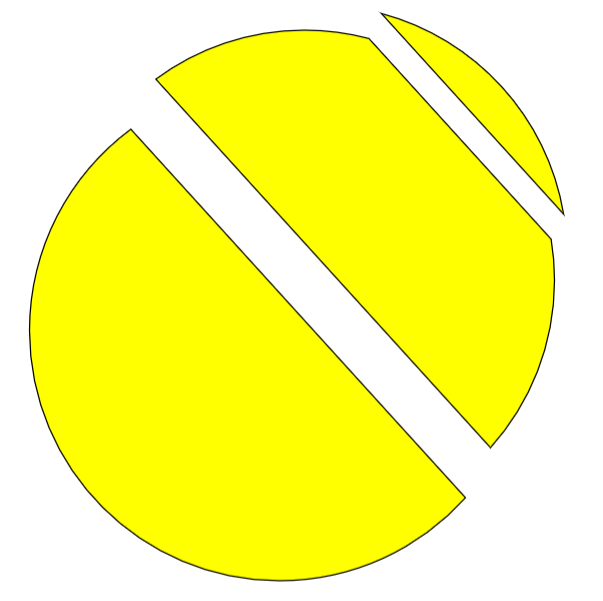
I searched for a solution in the paperJS to find out a decent solution which could help me do this slicing and I found that divide function from PaperJS can get the task done, but for multiple cuts I would have to create a function.
So as a solution I present that following code which I used to solve this problem.
var myCircle = new Path.Circle(new Point(300, 300), 200);
myCircle.fillColor = 'yellow';
myCircle.strokeColor = 'black';
function splitShape(shape,path)
{
var partsGroup = new Group();
partsGroup.addChild(shape);
// Looping all the Shapes.
for(var i=0; i<path.children.length; i++)
{
// Dividing the Path Multiple Times.
var tempParts = Array();
for (var x = 0; x < partsGroup.children.length; x++) {
if (path.children[i].intersects(partsGroup.children[x])) {
var result = partsGroup.children[x].divide(path.children[i], { insert: false, stroke: false, trace: false });
var clone = result.clone();
result.remove()
var group = new Group();
group.addChildren(clone.children);
for (var s = 0; s < group.children.length; s++) {
group.children[s].closed=true;
tempParts.push(group.children[s]);
}
}
else {
tempParts.push(partsGroup.children[x]);
}
} // end for
partsGroup.removeChildren();
for(var y=0; y<tempParts.length;y++)
{
partsGroup.addChild(tempParts[y],{insert:false})
}
} // end for
return partsGroup;
}
var path = new CompoundPath({
children: [
new Path.Line([100, 50], [600, 600]),
new Path.Line([300, 50], [800, 600])
],
fillColor: 'black',
selected: true,
});
var partsGroup = splitShape(myCircle,path)
// Code to Move the Pieces Apart.
for (var x = 0; x < partsGroup.children.length; x++) {
partsGroup.children[x].position = partsGroup.children[x].position + [-10*x,20*x]
}
path.selected=false;
Leave a Reply
You must be logged in to post a comment.