To draw a Ellipsis in Paper.js is quite simple and straightforward. You do not need to write a script to that.
Problem
But in my recent project I faced a situation where I had to draw a ellipsis kind of shape within 4 points (In my case they form a Rectangle.)
The problem with the PaperJS way of creating Ellipsis is that we can create a Ellipsis by giving it a Rectangle. But the way rectangles are drawn is only by providing two points which automatically calculates the other two sides. But it assumes that the Rectangle will form at 90 degrees on the Canvas.
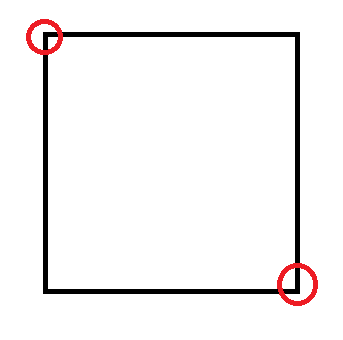
But when you are drawing a Rotated Rectangle it is not possible to recreate without knowing the Angle of Rotation.
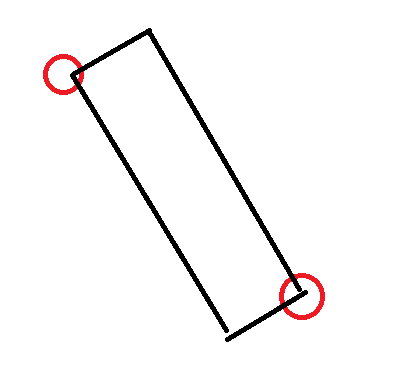
When I am creating a Rectangle with 4 points it cannot always be at a right angle on the Coordinate system.
To draw such a diagram I created a small function. which produces the required output.

Solution
var rectanglePoints = [[10, 10], [400,10], [400, 500],[10,500]];
var path = new Path({
segments: rectanglePoints,
fillColor: 'grey',
closed: true
});
var midPoints = Array();
for(var i=0;i<rectanglePoints.length-1;i++)
{
var first = rectanglePoints[i];
var second = rectanglePoints[i+1];
var x = (first[0]+second[0])/2;
var y = (first[1]+second[1])/2;
midPoints.push([x,y]);
}
var first = rectanglePoints[rectanglePoints.length-1];
var second = rectanglePoints[0];
var x = (first[0]+second[0])/2;
var y = (first[1]+second[1])/2;
midPoints.push([x,y]);
midPoints.unshift([x,y])
var path2 = new Path();
path2.strokeColor='red'
path2.strokeWidth= 1,
path2.addSegment(midPoints[0])
for(var i=0;i<rectanglePoints.length;i++)
{
path2.quadraticCurveTo(rectanglePoints[i], midPoints[i+1])
}
path2.fillColor = 'pink'
In this function, I use Quadratic Curve to create the Ellipsis.
Leave a Reply
You must be logged in to post a comment.